Constructors In Java
We are examining Java constructors with examples, so that Java developers can satisfy their demands more effectively.
Here are some key points to keep in mind when writing constructors:
- It is mandatory that the constructor(s) of a class have the same name as the class it belongs to.
- Java constructors cannot be abstract, final, static, or synchronized.
- To control access to constructors, access modifiers can be used in their declarations.
Java Constructors
Whenever we say Java constructors, we are talking about special methods for initializing objects.
The constructor is called when an object of a class is created.
It can be used to set initial values for object attributes.
Syntax:
class ClassName {
ClassName() {
}
}
Creating a constructor is as simple as below examples:
// Create a Main class
public class Main {
String mrx_name; // Initialize a variable mrx_name in Main class
// For the Main class, create a constructor
public Main() {
mrx_name = "James Gosling"; // Assign a value to the class attribute mrx_name
}
public static void main(String[] args) {
Main my_Object = new Main(); // The constructor will be called when you create an object of class Main.
System.out.println("Java Founder: "+my_Object.mrx_name); // The value of mrx_name should be printed
}
// Outputs James Gosling
}
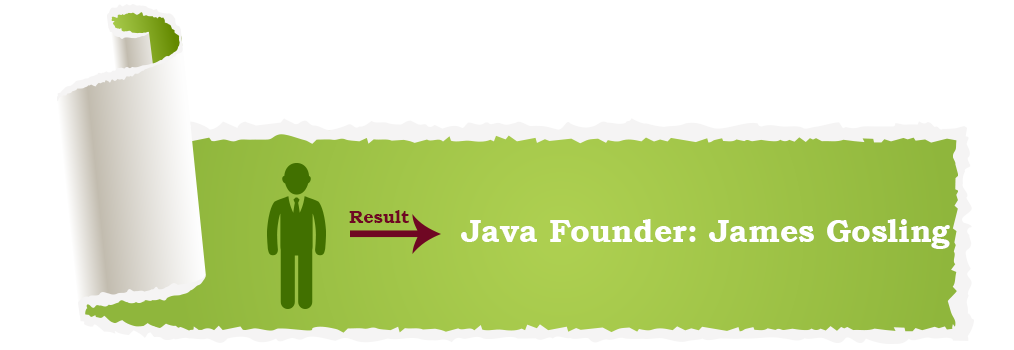
// Create a Main class
public class Main {
float mrx; // Initialize a 3 float variables (mrx,ample,result_mrx_ample) in Main class
float ample;
float result_mrx_ample;
// For the Main class,to access the attributes create a constructor
public Main() {
// Assign values to the class attribute
mrx=5.23f;
ample=4.35f;
result_mrx_ample=mrx+ample;
}
public static void main(String[] args) {
Main my_Object = new Main(); // The constructor will be automatically called when you create an object of class Main.
System.out.println("Sum of floats: "+my_Object.result_mrx_ample); // The sum of two float numbers should be printed
}
// Outputs Sum of floats: 9.58
}
You need to ensure that the constructor’s name matches the name of the class, and should not contain a return type such as (int) .
When an object is created, the constructor is called.As a default, every class has a constructor: if you don’t create it yourself, Java will create one for you.
If you do this, you will not be able to set initial values for object attributes.
Constructor Parameters
Parameters can also be passed to constructors, which are used to initialize attributes.
A parameter of type double mrx is added to the constructor in the following example.
Inside the constructor we set ample to mrx (ample=mrx).
When we call the constructor, we pass a parameter to the constructor (5.62341), which will set the value of mrx to 5.62341:
public class Main {
double mrx;
public Main(double ample) {
mrx=ample;
}
public static void main(String[] args) {
Main my_Object = new Main(5.62341d);
System.out.println("mrx = "+my_Object.mrx);
}
// Outputs mrx = 5.62341
}
public class Main {
String name;
public Main(String ample_name) {
name=ample_name;
}
public static void main(String[] args) {
Main my_Object = new Main("James Gosling");
System.out.println("Java Founder= "+my_Object.name);
}
// Outputs Java Founder = James Gosling
}
It is possible to have as many parameters as you like:
public class Main {
int mrx_year;
String ample_founder_name;
public Main(String ample,int mrx){
ample_founder_name=ample;
mrx_year=mrx;
}
public static void main(String[] args) {
Main my_object=new Main("James Gosling",1995);
System.out.println("Java Language was founded by: "+my_object.ample_founder_name+" in year: "+my_object.mrx_year);
}
// Output: Java Language was founded by: James Gosling in year: 1995
}
public class Main {
int mrx_num;
int ample_num;
public Main(int mrx,int ample){
mrx_num=ample;
ample_num=mrx;
}
public static void main(String[] args) {
Main my_object=new Main(15,6);
System.out.println("Product of two numbers: "+(my_object.mrx_num*my_object.ample_num));
}
// Output: Product of two numbers: 90
}
Java Constructors vs Methods: What’s the Difference?
- A constructor in Java must have the same name as the class in which it is created. However, it is not necessary for a method to have the same name.
- A constructor does not return a type, whereas a method(s) does if it returns nothing.
- When an object is created, a constructor is called only once, while a method(s) can be called as many times as needed.
Now that you know what constructors are and how to use them, you will be able to create your own classes.