Understanding F# Data Types
We are going to explore F# Data types in this article, including the most basic types, such as numbers and strings, as well as the different types of numbers and strings in F#.
Here, we will take a closer look at how these types can be used to represent and manipulate data in various ways.
The F# programming language was created with the intent of being highly expressive and concise. This makes it the ideal language for developing complex applications that rely heavily on data to process.
As one of F#’s strongest features, the powerful type system is one of the most important features. With an abundance of types and constructs, F# provides a robust set of options for working with data in a type-safe and efficient manner.
After finishing this article, you should have a good idea of what the F# Data types are and how you can use them effectively in the development of your own F# applications.
F# Data Types
F# offers a set of data types which can be classified in the following ways:
- Integral types
- Floating point types
- Text types
- Other types
Integral Data Type
Here is a table that includes the integral data types that are available in F#.
Basically, these are types of data that can be expressed as integers.
F# Types | Size | Range | Examples | Remarks |
sbyte | 1 byte | -128 to 127 | 42y
-11y |
8-bit signed integer |
byte | 1 byte | 0 to 255 | 6uy
5uy |
8-bit unsigned integer |
int16 | 2 bytes | -32768 to 32767 | 4s
9s |
16-bit signed integer |
uint16 | 2 bytes | 0 to 65,535 | 42us
200us |
16-bit unsigned integer |
int/int32 | 4 bytes | -2,147,483,648 to 2,147,483,647 | 35
44 |
32-bit signed integer |
uint32 | 4 bytes | 0 to 4,294,967,295 | 212u
504u |
32-bit unsigned integer |
int64 | 8 bytes | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 | 7878L
4548L |
64-bit signed integer |
uint64 | 8 bytes | 0 to 18,446,744,073,709,551,615 | 454521UL
544000UL |
64-bit unsigned integer |
bigint | At least 4 bytes | any integer | 42I
149999999999 999999999999 9999999I |
arbitrary precision integer |
Example: 
Floating Point Data Types
A list of F# floating point data types can be found in the following table.
F# Types | Size | Range | Examples | Remarks |
float32 | 4 bytes | “±1.5e-45 to ±3.4e38” | 35.0F
45.0F |
7 significant digits, 32-bit signed floating point number |
float | 8 bytes | “±5.0e-324 to ±1.7e308” | 35.0
45.0 |
15-16 significant digits in a 64-bit signed floating point number |
decimal | 16 bytes | “±1.0e-28 to ±7.9e2” | 445.0M
1546.0M |
It has 28-29 significant digits and is signed in 128 bits |
BigRational | At least 4 bytes | Any rational number. | 78N
1315N |
Random rational number. If you want to use this type, you need to refer to the FSharp.PowerPack.dll library. |
Example: 
Example
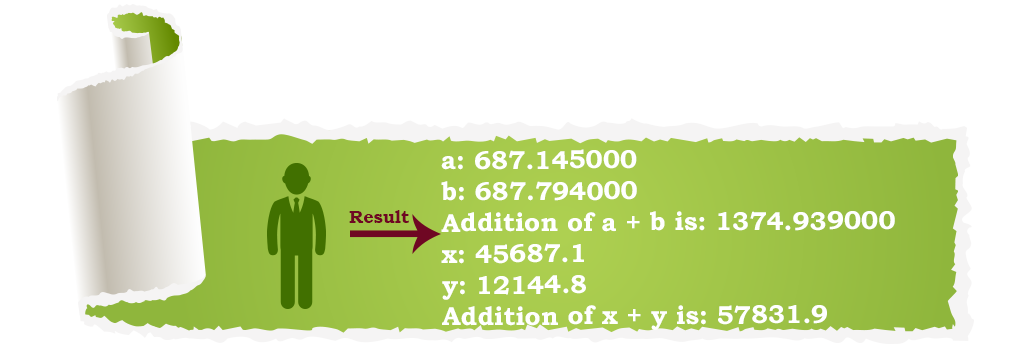
Example: 
a: 687.145000 b: 687.794000 Multiplication of a * b is: 472614.200000 x: 45687.1 y: 12144.8 Multiplication of x * y is: 5.54859e+08
F# Data Types – Text
F# Types | Size | Range | Examples | Remarks |
char | 2 bytes | U+0000 to U+ffff | x | Single unicode characters |
string | 20 + (2 * strings length) bytes | 0 to about 2 billion characters | “Hello”
“World” |
Unicode text |
Example: 
Output
Choice: a Name: John Wick Website: mrexamples
Example: 
Choice: a Hello from mrexamples
Other Data Types
This table provides some information on some of the other data types available in F#.
F# Types | Size | Range | Examples | Remarks |
bool | 1 byte | True or false are the only two possible values that can be assigned to it | true
false |
This function stores boolean values |
Example
True Value: true False Value: false