F# if-else if-elif-else Conditions
In F# if then else are a powerful conditions for controlling program flow and executing code selectively based on the evaluation of a given expression.
In order for a F# If then else conditions structure to be implemented, you must specify one or more conditions that need to be tested or evaluated by the program.
A description of the condition should be accompanied by at least one statement to be executed upon determining whether the condition is true. Optionally, there should be other statements to be executed upon determining whether the condition is false.
In this post we will cover the F# if/then, the if/then/else, the if/then/elif/else expressions, and the nested if expressions, which are some of the most commonly used constructs in F#.
F# if-else Statement
F# If/else statements are another most common decision-making construct.
It enables you to create code that makes a decision when a given condition is met as well as handle scenarios when the condition is not met.
Syntax
if expr then expr else expr
Below is an example of checking if age is less than 17 so print if statement otherwise print else statement:
Example: 
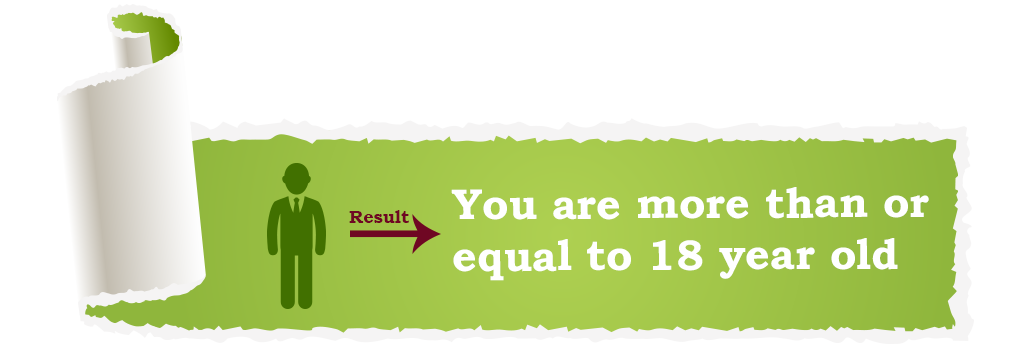
Example Explanation
Above F# code declares a variable named ‘age’ of type ‘int32’ and assigns it a value of 27.
The next line uses an ‘if’ statement to check if the value of ‘age’ is less than 18. If the condition is true, then the block of code inside the ‘then’ keyword is executed. In this case, the block of code prints a message to the console using the ‘printfn’ function. The message includes the value of ‘age’ as part of the string output.
However, since the condition is false (since age is greater than or equal to 18), the code executes the block of code following the ‘else’ keyword. This block of code also prints a message to the console using the ‘printfn’ function. The message simply states that the person’s age is more than or equal to 18.
Overall, this code demonstrates the use of an ‘if/else’ statement to perform a basic decision-making task in F#. The code prints different messages to the console based on the value of ‘age’.
F# if-then Statement
There are a number of fundamental constructs in F# that can be used to make decisions, and one of the most fundamental is the ‘if/then’ statement.
In F#, a block of code can be executed based on what conditions need to be met in the if/then statement.
Syntax
//simple if if expr then expr
By using the if/then condition we are printing that the user is less than 18 years old in the example below:
Example: 
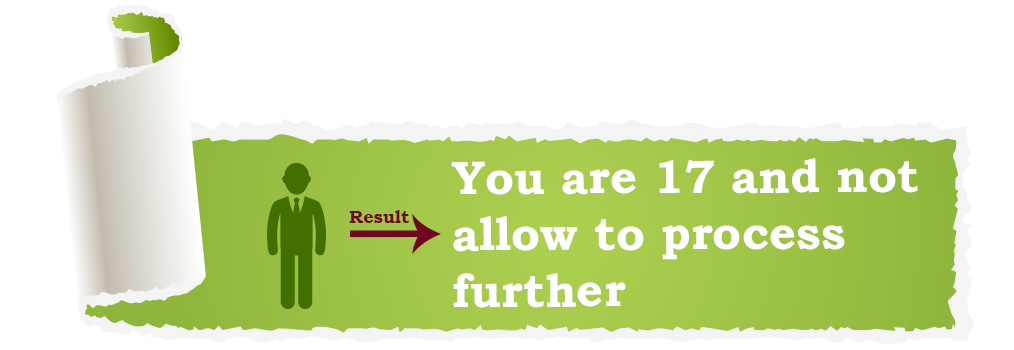
Example Explanation
This F# example declares a variable named ‘age’ of type ‘int32’ and assigns it a value of 17.
The next line uses an ‘if‘ statement to check if ‘age’ is less than 18. If the condition is true, the block of code inside the ‘then’ keyword is executed. In this case, the block of code simply prints a message to the console using the ‘printfn‘ function. The message includes the value of ‘age’ as part of the string output.
The message printed to the console states that the person’s age is below 18 and they are not allowed to process further. This implies that the code is likely related to age restrictions for a particular process or activity.
F# if-elif-else Statement
F# if-elif-else statement can be used in complex scenarios when making decisions.
You can write code to evaluate multiple conditions sequentially, handle scenarios where a given condition is not met, and make a choice based on the condition.
Syntax
if expr then expr elif expr then expr elif expr then expr ... else expr
Below is an example of evaluating multiple conditions on the basis of multiple age groups:
Example: 
Example Explanation
Above example uses F# if statement to check a Boolean condition based on the value of a variable “age” which is declared as an integer with a value of 29.
Then proceeds to execute a series of conditional statements based on the value of “age”.
- If “age” is less than 18, the code will print a message stating the person’s age.
- If “age” is greater than or equal to 18 AND less than 30, the code will print a message saying “You are young.”
- If “age” is greater than or equal to 30 AND less than 55, the code will print a message saying “You are bigger than young.”
- If “age” is greater than or equal to 55, the code will print a message saying “You are old.”
- If none of these conditions are met, the code will print a message asking the user to enter their correct age.
In this case, since the value of “age” is 29, the code will execute the second conditional statement and print “You are young.”
F# Nested if Statement
Nested if is among the most commonly used programming constructs in many languages, including F#, to create nested if statements.
In F#, you can use nested if statements to specify a sequence of conditions to check. Once a condition is evaluated, the next condition will only be evaluated if the previous one failed.
Syntax
if expr then expr if expr then expr else expr else expr
Example below check that, if condition is true then it will run nested if otherwise else will be execute:
Example: 
Example Explanation
Above example is a nested if statement to check a boolean condition based on the value of a variable “age”, which is declared as an integer with a value of 29.
The first if statement checks if the value of “age” is greater than 18. If it is, the code inside the if block will be executed. In this case, it will print the message “You are more than 18 year old” and then proceed to another nested if statement.
The nested if statement checks if the value of “age” is between 18 and 30, inclusive. If it is, the code inside the if block will be executed. In this case, it will print the message “And, You are young!”.
If “age” is not between 18 and 30, the code inside the else block will be executed, which will print the message “And, You are old!”.
If the value of “age” is not greater than 18, the code inside the else block of the outer if statement will be executed, which will print the message “Please enter your correct age.”
In this case, since the value of “age” is 29 and it satisfies both conditions, the code will execute the nested if statement and print “You are more than 18 year old” and “And, You are young!”.
You can help others learn about the power and flexible nature of F# if conditions by sharing our article on social media below. This will enable them to create dynamic and interactive web applications.