F# Strings
F# strings are represented as Unicode character arrays, and F# comes with a very rich set of functions that allow you to work with strings such as concatenation, substring extraction, regular expression matching, and more.
F# includes a number of useful features, such as pattern matching and type inference, which make it both easy and efficient to work with F# strings.
This article will introduce some of F# string manipulation features, including Unicode support, string concatenation, string formatting, parsing, and matched regular expressions to match patterns.
F# String Literals
Literals in string form are delimited by quotation marks “ to indicate that they are strings.
A number of special characters are used for specific purposes, such as newlines, tabs etc.
Using the backslash \ character, they are encoded. Backslashes are used as part of the escape sequence, along with its related character.
Below is a table of F#’s escape sequences.
Characters | Escape sequences |
Backspace | \b |
Newline | \n |
Carriage return | \r |
Tab | \t |
Backslash | \\ |
Quotation mark | \” |
Apostrophe | \’ |
Unicode character | \uXXXX or \UXXXXXXXX (where X indicates a hexadecimal digit) |
Ignore Escape Sequence
Compilers can ignore escape sequences in two different ways, as shown below:
- By using the @ symbol.
- Put a triple quote “‘ around the string.
The @ symbol precedes a string literal, which is called a verbatim string. This way, all escape sequences in the string are ignored, with the exception of two quotation mark characters being interpreted as a single quotation mark character.
Whenever a string is enclosed in triple quotation marks, all escape sequences, including the double quotation marks, are ignored, which means that all escape sequences are ignored.
Example
Using the following example, we will demonstrate how to use this technique when dealing with structures containing embedded quotation marks in XML or other languages:
Example: 
The output of the following example can be seen as follows:
<user firstname = "Jennifer" lastname = "Anniston">
F# Strings Operators
Strings are the sequences of characters that make up a program.
There are many basic operators on strings that can be used to manipulate strings in programming languages.
Among the most common basic operators that can be used on strings are the following:
Value | Overview |
collect : (char → string) → string → string | The purpose of this function is to create a string whose characters are the result of appending a specified function to the characters in the input string and producing a new string. A concatenated string is created as a result. |
concat : string → seq<string> → string | By concatenating the strings with a separator, this method returns a new string which contains all of those strings combined. |
exists : (char → bool) → string → bool | The function determines whether any character in the string meets the predicate given by the user. |
forall : (char → bool) → string → bool | This function determines whether all characters in the string satisfy the predicate given in the input string. |
init : int → (int → string) → string | This method will create a new string which will contain characters created by applying a predefined function to each index and concatenating the resulting strings together to form the new string. |
iter : (char → unit) → string → unit | Each character in the string will be applied with the specified function. |
iteri : (int → char → unit) → string → unit | This function can be applied to each index of a character in a string as well as to the character itself according to a specification. |
iteri : (int → char → unit) → string → unit | A string’s length is returned by this method. |
map : (char → char) → string → string | In this function, the input string is converted into a new string with characters whose values are determined based on the application of a specified function to each value within the string. |
mapi : (int → char → char) → string → string | In this method, the characters in the input string are replaced with the results of applying a specified function to each of the characters and indexes within the input string to produce a new string. |
replicate : int → string → string | An instance of a string is concatenated with the specified number of instances of another string to return a string. |
Here are a few examples of how some of the above functionalities can be used in the following scenarios:
F# String.collect
String.collect is used to create a string from the input character string by applying a specified function to all the characters of that string to create a new string whose characters result from the application of that function. As a result of this, a string has been concatenated.
Example: 
The following is the output that you will receive once you have compiled and executed the program:
F# String.concat
Using String.concat, you can concatenate a sequence of strings with a separator and return a new string after concatenating the strings.
Example: 
The output of the example mentioned above is:
F# String.exists
In order to determine whether a substring exists in a given string, we can use the String.exists function.
Example: 
As ‘mrexamples’ is placed in the statement, the output will be:
F# String.forall
Using String.forall, you can test whether a condition holds for all characters within the string that you are given.
Example: 
‘MREXAMPLES’ is in uppercase, therefore the output will be as follows:
All characters in the message are uppercase.
F# String.init
Using String.init, you can construct a new string and apply a function to each index in order to generate a new string.
Example: 
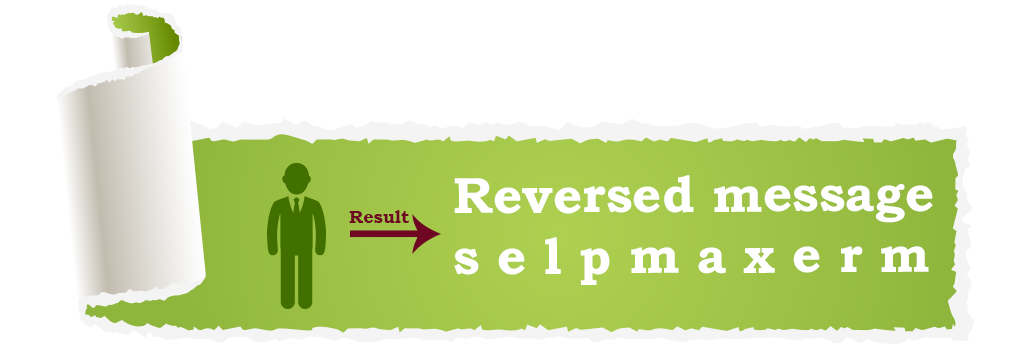
F# String.iter
If a string contains a series of characters, we can use String.iter to perform a specific action on each one.
Example: 
Following is the output of the above example to print every character of the string:
m r e x a m p l e s
F# String.iteri
A String.iteri can be used for iterating over every character in a string, as well as its index within the string.
Example: 
Here is an example which prints both the character that is being displayed as well as its position within the string:
Char 0 is 'm' Char 1 is 'r' Char 2 is 'e' Char 3 is 'x' Char 4 is 'a' Char 5 is 'm' Char 6 is 'p' Char 7 is 'l' Char 8 is 'e' Char 9 is 's'
F# String.length
The length of a string can be determined by using String.length, which returns an integer of all characters in a string.
Example: 
Above example prints the length of the message with the help of length function:
The lenght of the message is: 10
F# String.map
Using String.map, it is possible to apply a given function to every character in a string in order to create a new string with the transformed characters instead of just returning the original strings.
Example: 
Below is an example of the function map() to map string characters and transform instead of returning the original strings.
Original message: mrexamples! Reversed message: !selp!axerm
F# String.mapi
Using String.mapi, you are able to map a character of a string to a single value, based on the index of the character in the string.
Example: 
In the above example, the message will be transformed with uppercase characters on every even index:
Original message: mrexamples Modified message: MrExAmPlEs
F# String.replicate
The method String.replicate can be used to create a new string consisting of repeated characters or strings with a specified number of repetitions.
Example: 
Following example output the replicate of ‘s’ at the end of string
mrexamplesssss
Example Explanation
This F# code creates a new string s by using the String.replicate function to repeat the string “s” five times.
It then prints out a formatted string using printfn that includes the s string concatenated to the end of mrexample.
Here’s a step-by-step explanation of what happens:
- String.replicate 5 s creates a new string by repeating the string “s” five times, resulting in the string sssss.
- let s = String.replicate 5 s binds the new string to the variable s.
- printfn “mrexample%s” s prints a formatted string to the console, where %s is a placeholder for the s string. The s string is then concatenated to the end of mrexample to produce the final output: mrexamplesssss.’