PHP foreach Loop – A Comprehensive Guide
In this article, we’ll explore how PHP foreach loop works and how it can be used in production environment.
With PHP foreach loop, You can easily iterate over arrays and other iterable data structures.
This loop automatically assigns the key and value of each array element to loop variables, making accessing and manipulating array data easy.
It helps you quickly and easily process your data, whether you’re working with a simple array or a multidimensional array.
PHP foreach Loop
With the use of the foreach loop, each key/value pair in an array is looped through one by one.
It is an ideal choice to use a foreach loop when you don’t know the exact number of elements in an array or object in advance.
Syntax
foreach ($array as $value) { //The code that needs to be executed }
In each iteration of the loop, the current array element’s value is assigned to the variable $value, then the pointer to the next array element is moved by one, until the array reaches the end of the array.
Below is an example that outputs the contents of the array ($flower):
Example: 
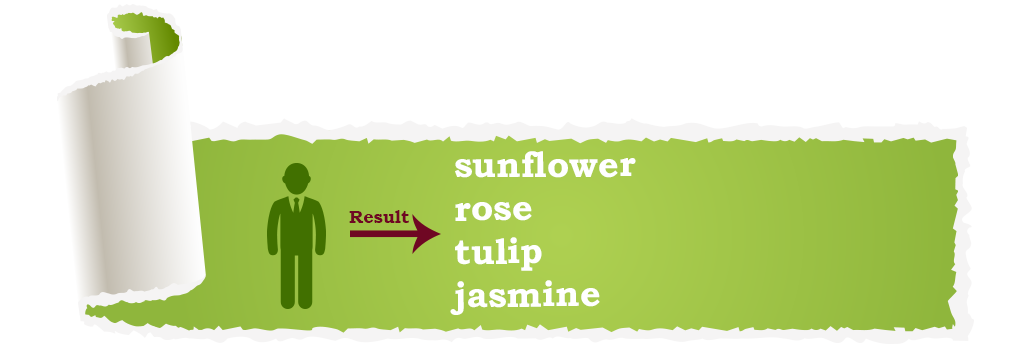
Example: 
Example: 
Example Explanation
Above examples code defines an associative array called $salary, which contains the salaries of three individuals – Henry, Rachel, and Louis.
The keys of the array are the names of the individuals, and the values are their respective salaries.
Then we have used a foreach loop to iterate through the array. In each iteration of the loop, the key of the current array element is assigned to the loop variable $a, and the value of the current array element is assigned to the loop variable $value.
Inside the loop, the code prints the name of the individual (stored in $a), followed by their salary (stored in $value), and a line break (<br>). The output of this code would be a list of the names and salaries of each individual in the $salary array.
Our upcoming article on PHP Arrays will give you a better understanding of arrays.