Python Step-by-Step Guide
Python will be discussed in this post to get you started, hoping to fulfill your learning needs.
Python Installation:
The Python programming language comes pre-installed on many PCs and Macs.
The easiest way to determine if you have Python installed on your Windows PC is to search for Python in the start bar or to run the following command on the windows command line (cmd.exe):
On both Linux and Mac operating systems, you can check whether you have Python installed by opening the terminal and typing the following code:
Python Installation For Windows:
You can easily install Python on Windows and start using it right away. There are only three steps to the installation process:
- Download Python for Windows.
- Execute the executable installer.
- Python should be added to the PATH environment variable.
The official Python executable installer must be downloaded in order to install Python. You will then need to run this installer and complete the installation process.
Finally, you can use Python from the command line by configuring the PATH variable. Python is available in a variety of versions. This article recommends installing Python 3.7.3, the most stable version.
Step 1: Download Python:
- Visit the official Python for Windows website.
- Make sure to choose the stable Python 3 release. Our example uses Python 3.7.3.
- Download the Windows x86 executable installer if you are using 32-bit Windows. If your Windows installation is a 64-bit system, then you should download the Windows 86-64 executable installer.
Step 2: Execute the executable installer:
- Run the Python installer after downloading it.
- Make sure the Install launcher for all users check box is checked. In addition, you may include Python 3.7 in the execution path by checking the Add Python 3.7 to path check box.
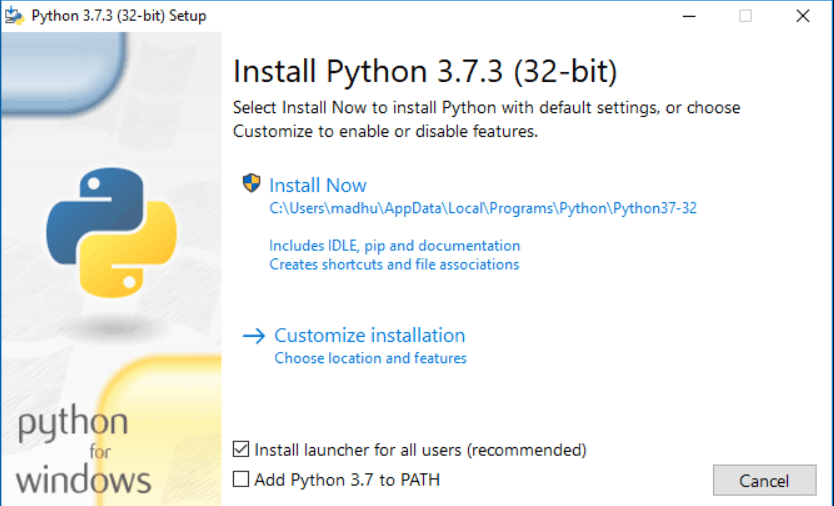
- Make sure Customize installation is selected. Click the check boxes next to the optional features as shown in below Image:
- Documentation.
- pip.
- tcl/tk and IDLE (to install tkinter and IDLE).
- Python test suite (to install the standard library test suite of Python).
- Install the global launcher for `.py` files. This makes it easier to start Python.
- Install for all users.
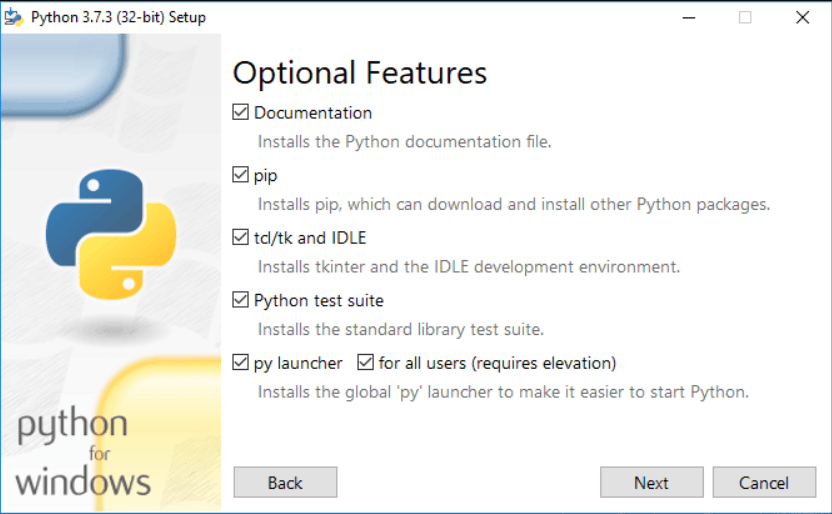
- Click Next: This opens Advanced options. Check the boxes below:
- Select Install for all users.
- Associate files with Python. (requires the py launcher)
- Create shortcuts for installed applications.
- Add Python to the environment variables check box.
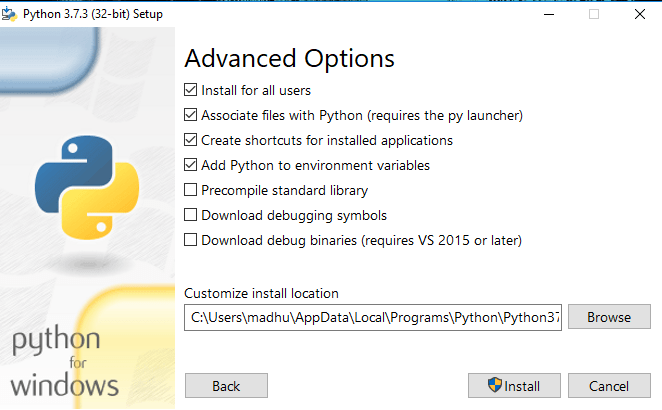
Take note of the Python installation directory. Install the Advanced options by clicking Install.
- A Python Setup Successful window will appear after the installation is completed.
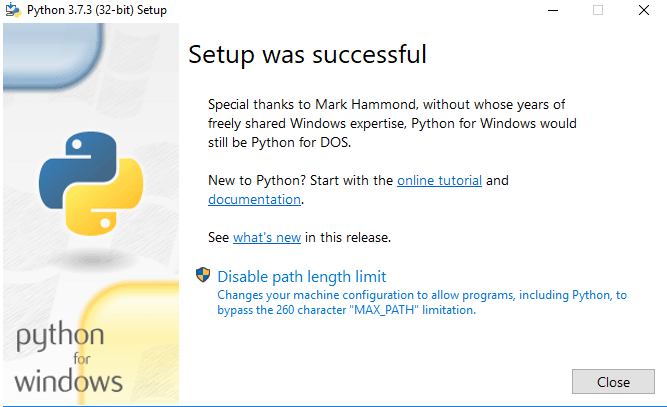
Step 3: Integrating Python with environmental variables:
The final (optional) step in the Python for Windows installation process is to set the Python Path in the System Environment Variables.
Python is accessed via the command line in this step.
You can skip this step if you added Python to the environment variables at the time of setting the Advanced options during installation. Otherwise, this step must be performed manually.
- Go to the Start menu and search for “advanced system settings”.
- Click on “View advanced system settings”.
- Select the “Advanced” tab in the “System Properties” window, then click on “Environment Variables”.
- You will need to locate the Python installation directory on your computer.
You will be able to find Python in the following locations if you followed the instructions exactly as described above:
- C:\Program Files (x86)\Python37-32: for 32-bit installation
- C:\Program Files\Python37-32: for 64-bit installation
If you installed a different version of Python, the folder name may differ from “Python37-32”.
- Find a folder that starts with Python.
PATH variable should be updated as given in below example image:
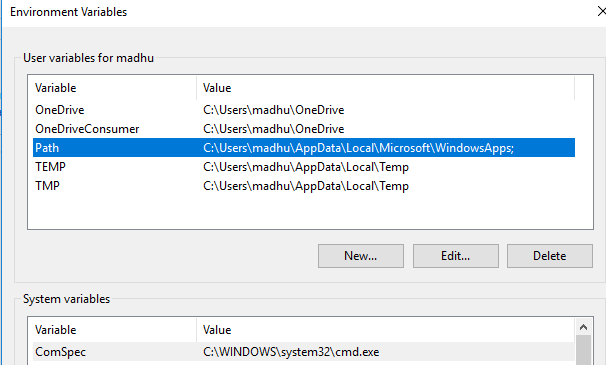

Step 4: Verify Python Installation:
Installing Python can either be verified through the command line or through the IDLE application that gets installed along with it. Start by typing “python” at the command prompt.
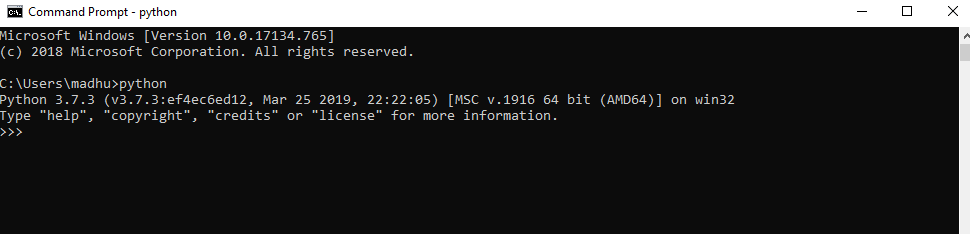
You can see that having successfully installed Python 3.7.3 on Windows, you are now ready to use it. To get started with Python coding, use the Integrated Development Environment (IDLE).
Python Installation For Linux Operating System:
We have prepared a separate topic for step-by-step instructions on installing Python on a Linux operating system.
Python Installation For Mac Operating System:
An additional topic will be covered in order to assist you in installing Python on a Mac operating system.
Python Quickstart
A Python programmer writes python files (.py) using a text editor, then runs them in the Python interpreter using the python interpreter.
Running a Python file on the command line looks like this:
In above example, “pythontutorial.py” is the name of the Python file you are using.
Using any text editor, let’s create our first Python file, myfirstpythonfile.py.
myfirstpythonfile.py
Example
The rest is simple. Make sure you save your file. Using the command line, navigate to the directory where you have saved your file, and run:
This should be the output:
Your first Python program has been written and executed. Congratulations!
Python in Command Line:
Whenever you want to test a short piece of Python code, you don’t have to write it to a file. Python can be run from the command line by itself, making this possible.
Run the following command on Windows, Mac, or Linux:
If the “python” command doesn’t work, try “py”:
You can then write any Python code, including our myfirstpythonfile example from earlier:
Python 3.6.4 (v3.6.4:d48eceb, Nov 30 2022, 05:04:40) [MSC v.1900 32 bit (Intel)] on win32
Type “help”, “copyright”, “credits” or “license” for more information.
>>> print(“This is your first code in python, Congrats.”)
It will display “This is your first code in python, Congrats.” in the command line:
Python 3.6.4 (v3.6.4:d48eceb, Nov 30 2022, 05:05:18) [MSC v.1900 32 bit (Intel)] on win32
Type “help”, “copyright”, “credits” or “license” for more information.
>>> print(“This is your first code in python, Congrats.”)
This is your first code in python, Congrats.
The Python command line interface can be quit by typing the following when you are done using it:
Is Python Easy ?
Yes, Python is widely regarded as an easy programming language to learn and use, especially for beginners. Here are a few reasons why Python is considered easy:
- Python has a clean and readable syntax that resembles plain English. The code is structured using indentation, which enhances code readability. This makes it easier for beginners to understand and write code.
- Python’s syntax is designed to be simple and concise. It requires fewer lines of code compared to other languages, making it easier to write and maintain. Python’s focus on simplicity reduces the learning curve for beginners.
- Python has a wealth of comprehensive documentation and a large online community that provides tutorials, guides, and answers to common questions. These resources make it easier for beginners to find assistance and learn the language at their own pace.
- Python has a vast and active community of developers who are willing to help and share their knowledge. This community offers support through online forums, discussion groups, and social media platforms. Having a supportive community is valuable for beginners as they can get guidance and advice from experienced developers.
- Python is a versatile language that can be used for a wide range of applications, including web development, data analysis, scientific computing, machine learning, and more. This versatility allows beginners to choose the domain they are interested in and start building projects relatively quickly.
- Python provides built-in data structures, such as lists, dictionaries, and sets, which simplify common programming tasks. Additionally, Python has a vast collection of libraries and frameworks that offer pre-built functionality, reducing the need to write code from scratch. These libraries make it easier to handle complex tasks and speed up development.