Python Strings Format Methods And Types
We will explain Python strings format and its types in this chapter with examples to meet your learning objectives.
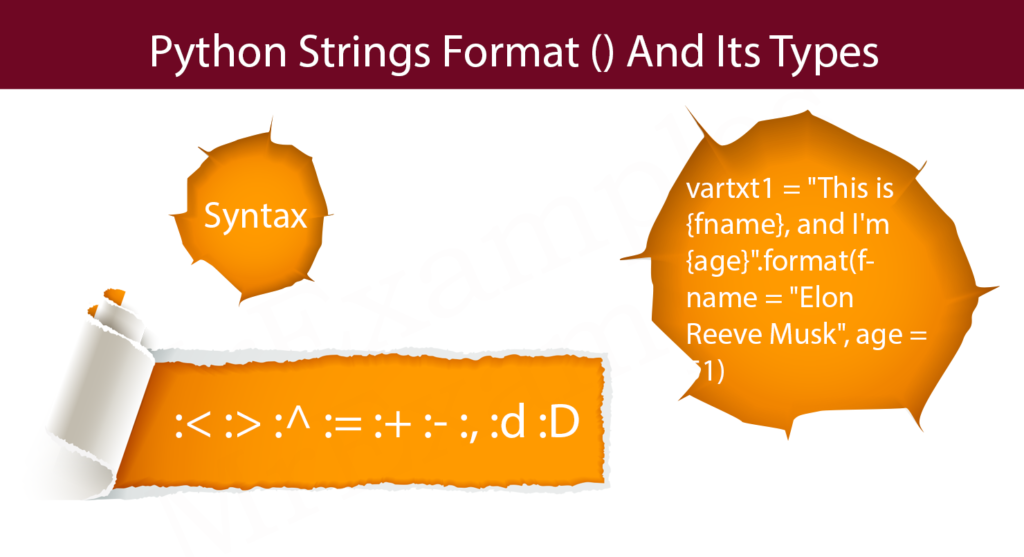
Python Strings Format – Definition and Usage
Using curly brackets, we define the placeholder: {}. In the Placeholder section below, you can learn more about the placeholders.
Strings and numbers cannot be combined like this in Python Variables:
What Is The Format Of a String In Python?
When we talk about Python strings format then in Python, a string is a sequence of characters enclosed in either single quotes (‘ ‘) or double quotes (” “). The choice between single or double quotes is up to the programmer, and both are used interchangeably.
Here are a few examples of string literals in Python:
name = ‘John’ message = “Hello, world!” sentence = “I’m learning Python.”
Python also supports triple quotes (”’ ”’) or (“”” “””) to represent multiline strings. Triple quotes allow you to include line breaks and preserve the formatting of the text. Here’s an example:
paragraph = ”’This is a multiline string. It spans multiple lines and preserves the line breaks and formatting.”’
In addition to the basic string literals, Python provides various string manipulation methods and features that allow you to work with strings efficiently.
What Is .2f Python Format?
In Python, the .2f format specifier is used to format floating-point numbers as strings with two decimal places. This format specifier is part of the Python strings format syntax in Python.
When using the .2f format specifier, the number will be rounded to two decimal places and displayed with two digits after the decimal point. If the number has fewer than two decimal places, it will be padded with zeros.
Here’s an example that demonstrates the usage of the .2f format specifier:
number = 3.14159 formatted_number = “{:.2f}”.format(number) print(formatted_number)
Output:
3.14
In this example, the variable number contains the value 3.14159. By applying the .2f format specifier within the format() method and using the “{:.2f}” format string, the number is formatted to have two decimal places. The resulting formatted number is then printed, which outputs 3.14.
You can also achieve the same result using f-strings, which is a more concise and preferred way of string formatting in Python 3.6 and above:
number = 3.14159 formatted_number = f”{number:.2f}” print(formatted_number)
The output will be the same as before: 3.14.
What Is %d In String Format?
In Python, the %d format specifier is used to format integers as strings within the context of the old-style string formatting method. It allows you to insert integer values into a string when it comes to Python strings format.
Here’s an example that demonstrates the usage of %d in string formatting:
age = 25 message = “I am %d years old.” % age print(message)
Output:
I am 25 years old.
In this example, the integer value age is inserted into the string using %d. The % operator is used to combine the format string with the value to be formatted. The resulting string is then printed.
Note that the %d format specifier only works with integers. If you try to use it with a non-integer value, you will encounter a TypeError.
It’s worth mentioning that the old-style string formatting method using % is considered legacy and has been replaced by more modern string formatting options, such as the .format() method or f-strings. These newer methods provide more flexibility and readability. Here’s an equivalent example using f-strings:
age = 25 message = f”I am {age} years old.” print(message)
The output will be the same: I am 25 years old.
What Does %% s Mean In Python?
In Python, %%s is not a valid format specifier or syntax. It appears to be an incorrect use of string formatting.
In Python, the standard format specifier for inserting a string into a formatted string is %s. The %s format specifier is used to represent a placeholder for a string value as it comes to Python strings format.
Here’s an example of using %s to format a string:
name = “Alice” message = “Hello, %s!” % name print(message)
Output:
Hello, Alice!
In this example, %s is used as a placeholder in the format string. The value of the variable name is inserted into the string using the % operator and %s format specifier.
If you encountered %%s in code, it may be a typo or an error. Double percent (%%) is used as an escape sequence to represent a literal percent sign in string formatting. For example, “%%s” would be used to print the string “%s” as a literal string, without any value substitution in Python strings format.
What Is ___ str __ In Python?
When we discuss Python strings format, then in Python, __str__ is a special method that you can define within a class to provide a string representation of an object. It is invoked when you use the str() function or the print() function on an instance of the class.
When you define the __str__ method in a class, you can customize the string representation of objects of that class to provide meaningful information. This method should return a string that represents the object.
Here’s an example that demonstrates the usage of __str__:
class Person: def __init__(self, name, age): self.name = name self.age = age def __str__(self): return f”Person(name='{self.name}’, age={self.age})” person = Person(“Alice”, 25) print(person)
Output:
Person(name=’Alice’, age=25)
In this example, the Person class defines the __str__ method. The method returns a formatted string representation of the object, including the name and age attributes. When the print() function is called with an instance of the Person class, the __str__ method is automatically invoked to provide the string representation of the object as it comes to Python strings format.
By defining the __str__ method, you can control how your objects are displayed as strings, making it easier to understand and debug your code.
What Does \\ S+ S+ Mean In Python?
In Python, the pattern \\s+ is a regular expression pattern that matches one or more whitespace characters.
Let’s break down the pattern:
- \\ is an escape sequence that represents a single backslash character \. In regular expressions, a backslash is used to escape special characters and give them their literal meaning.
- s is a special character in regular expressions that represents any whitespace character, such as spaces, tabs, or line breaks.
- + is a quantifier in regular expressions that specifies that the preceding pattern (in this case, \s) should match one or more times consecutively.
When combined, \\s+ matches one or more whitespace characters.
Here’s an example that demonstrates the usage of this pattern:
import re text = “Hello world” matches = re.findall(r”\s+”, text) print(matches)
Output:
[‘ ‘]
In this example, the re.findall() function is used to find all occurrences of one or more whitespace characters in the text string. The regular expression pattern r”\s+” is passed as the first argument to re.findall(). As a result, it returns a list containing the match found, which is the sequence of spaces in the string.
Regular expressions are powerful tools for pattern matching and text manipulation. They provide a concise way to specify complex patterns and search for matches within strings. The re module in Python provides various functions and methods for working with regular expressions as it comes to Python strings format.
What Does ___ Mean In Python?
In Python, the underscores (___) can have different meanings depending on their context. Let’s explore a few common use cases:
Placeholder Variables:
Underscores are often used as placeholder variables when you don’t intend to use the value. It is a convention to use a single underscore (_) or multiple underscores (__, ___, etc.) for such variables. For example:
for _ in range(5): print(“Hello”)
In this case, the loop variable _ is used as a placeholder. It indicates that the loop variable is not going to be used within the loop body.
Name Mangling:
Double underscores (__) at the beginning of an identifier trigger name mangling in class definitions. It is used to make a class attribute “private” or to avoid naming conflicts in subclasses. For example:
class MyClass: def __init__(self): self.__private_variable = 42 def __private_method(self): print(“This is a private method.”) obj = MyClass() print(obj._MyClass__private_variable) obj._MyClass__private_method()
Here, the double underscores in __private_variable and __private_method cause name mangling. The actual attribute names become _MyClass__private_variable and _MyClass__private_method. Although not truly private, it is a way to discourage accessing these attributes directly from outside the class.
Special Methods:
Double underscores are used to denote special methods in Python classes. These methods have a specific purpose and are invoked implicitly in certain situations. Examples include __init__ (constructor), __str__ (string representation), __len__ (length), and many more.
class MyClass: def __init__(self, value): self.value = value def __str__(self): return f”MyClass instance with value: {self.value}” obj = MyClass(42) print(obj) # Invokes __str__ method implicitly
In this example, the __init__ method is the constructor, and the __str__ method is invoked implicitly when the print() function is called on an instance of MyClass.
Underscores have different meanings depending on their usage, so it’s essential to understand the context in which they are used to interpret their purpose correctly as it comes to Python strings format.
What Is %s vs d In Python?
In Python, %s and %d are format specifiers used in string formatting to insert values into a formatted string. However, they are used for different types of data:
%s: The %s format specifier is used to represent a placeholder for a string value. It can be used to insert any type of data into a string, as it will automatically convert the value to its string representation. For example:
name = “Alice” age = 25 message = “My name is %s and I am %s years old.” % (name, age) print(message)
Output:
My name is Alice and I am 25 years old.
In this example, %s is used to insert the values of name and age into the string message.
%d: The %d format specifier is specifically used for inserting integer values into a formatted string. It is not suitable for inserting strings or other data types. For example:
quantity = 5 price = 10.5 total = quantity * price message = “The total cost is %d.” % total print(message)
Output:
The total cost is 52.
In this example, %d is used to insert the value of total (which is an integer) into the string message.
It’s important to note that the old-style string formatting using % and format specifiers (%s, %d, etc.) is considered legacy and has been replaced by more modern string formatting options, such as the .format() method and f-strings. These newer methods provide more flexibility and readability. Here’s an equivalent example using f-strings:
name = “Alice” age = 25 message = f”My name is {name} and I am {age} years old.” print(message)
The output will be the same: My name is Alice and I am 25 years old.
What Does ‘% d Mean In Python?
In Python, ‘% d’ is a format specifier used in string formatting to represent a placeholder for an integer value with optional space padding for positive numbers.
The format specifier ‘% d’ consists of the percent sign (%) followed by a space and the letter d.
Here’s an example that demonstrates the usage of ‘% d’:
number = 42 formatted_number = ‘% d’ % number print(formatted_number)
Output:
42
In this example, the integer value number is inserted into the formatted string using ‘% d’. The space before d indicates that positive numbers should be padded with a space character. Negative numbers will still be displayed with a leading minus sign.
If the number is positive, it will be preceded by a space character to align it with the negative numbers. If the number is negative, it will be displayed with a leading minus sign as usual.
Note that the ‘% d’ format specifier can also accept width and precision specifications, similar to other format specifiers. For example, ‘% 5d’ will pad positive numbers with spaces to a width of 5 characters.
It’s worth mentioning that the old-style string formatting using % and format specifiers (‘% d’, %s, etc.) is considered legacy and has been replaced by more modern string formatting options, such as the .format() method and f-strings. These newer methods provide more flexibility and readability. Here’s an equivalent example using f-strings:
number = 42 formatted_number = f'{number: d}’ print(formatted_number)
The output will be the same: 42.
What Is %R In Python?
In Python, %R is not a standard format specifier for string formatting. There is no built-in format specifier %R in Python’s string formatting syntax.
If you encountered %R in code, it might be a custom format specifier defined by a specific library or framework that extends Python’s string formatting capabilities. The meaning and behavior of %R would depend on the context in which it is used and the specific library or framework being used.
To provide a more accurate answer, please provide more context or details about where %R is used or the specific library or framework you are referring to.
What Does %C Do In Python?
In Python, %C is not a valid format specifier in the standard string formatting syntax. There is no built-in format specifier %C in Python’s string formatting.
If you encountered %C in code, it might be a custom format specifier defined by a specific library or framework that extends Python’s string formatting capabilities. The meaning and behavior of %C would depend on the context in which it is used and the specific library or framework being used.
To provide a more accurate answer, please provide more context or details about where %C is used or the specific library or framework you are referring to.
Python Strings Format Float
In Python, you can format a float value as a string using various methods. Here are a few commonly used approaches:
Using the format() method:
The format() method allows you to format a float value by specifying a format specifier within curly braces {}. The format specifier for floats is f. You can specify the desired number of decimal places using .n, where n represents the number of decimal places.
number = 3.14159 formatted_number = “{:.2f}”.format(number) print(formatted_number)
Output:
3.14
In this example, the format() method is used with the format string “{:.2f}” to format the number with two decimal places. The resulting formatted number is then printed.
Using f-strings:
F-strings provide a concise and more readable way to format strings in Python 3.6 and above. You can prefix the string with f and directly embed the float value within curly braces {}. Similar to the format() method, you can specify the desired number of decimal places using :.n.
Number = 3.14159 formatted_number = f”{number:.2f}” print(formatted_number)
The output will be the same: 3.14.
Using the % operator:
The % operator can be used for string formatting in Python, known as the old-style string formatting. For floats, the format specifier %f is used, followed by the desired number of decimal places using .n.
number = 3.14159 formatted_number = “%.2f” % number print(formatted_number)
The output will be the same: 3.14.
These are a few common methods to format a float as a string in Python. Choose the one that suits your preference and the requirements of your code.
Python Strings Format f
In Python, the f format specifier, also known as f-string or formatted string literal, allows you to easily format strings by embedding expressions directly within curly braces {}. The expressions are evaluated and their values are inserted into the string.
Here’s an example of using f-strings to format strings in Python:
name = “Alice” age = 25 formatted_string = f”My name is {name} and I am {age} years old.” print(formatted_string)
Output:
My name is Alice and I am 25 years old.
In this example, the variables name and age are directly embedded within the string using f-string syntax. The expressions {name} and {age} are evaluated and their values are inserted into the string when it is printed.
F-strings provide a concise and readable way to format strings, as they allow you to include expressions directly within the string declaration. You can also apply formatting options to the expressions within the curly braces. For example, you can specify the number of decimal places for a float value or control the width of an integer.
Here’s an example demonstrating additional formatting options using f-strings:
pi = 3.14159 formatted_number = f”The value of pi is approximately {pi:.2f}” print(formatted_number)
Output:
The value of pi is approximately 3.14
In this example, the expression {pi:.2f} formats the value of pi as a float with two decimal places.
F-strings offer a powerful and flexible way to format strings in Python, allowing you to easily incorporate variables and expressions directly within your string declarations.
Python f-String Format
In Python, f-strings (formatted string literals) provide a concise and convenient way to format strings. With f-strings, you can embed expressions and variables directly within curly braces {}.
To format values within f-strings, you can apply various formatting options. Here are some common formatting options you can use within f-strings:
Specifying Width and Alignment:
You can specify the width and alignment of the formatted value by adding a colon : followed by a format specification. For example:
name = “Alice” age = 25 formatted_string = f”Name: {name:>10} | Age: {age:03}” print(formatted_string)
Output:
Name: Alice | Age: 025
In this example, the format specification >10 ensures that the value of name is right-aligned within a field of width 10, padded with spaces if necessary. The format specification 03 ensures that the value of age is zero-padded to a width of 3.
Specifying Floating-Point Precision:
For floating-point values, you can specify the precision (number of decimal places) using the format specification .n. For example:
pi = 3.14159 formatted_number = f”Value of pi: {pi:.2f}” print(formatted_number)
Output:
Value of pi: 3.14
In this example, the format specification :.2f ensures that the value of pi is formatted as a float with two decimal places.
Formatting Numeric Representations:
You can format numeric values using additional format specifications. For example:
number = 42 formatted_number = f”Number: {number:b} {number:x}” print(formatted_number)
Output:
Number: 101010 2a
In this example, the format specifications :b and 😡 format the value of number as binary and hexadecimal representations, respectively.
Python f-String
In Python, f-strings (formatted string literals) provide a concise and expressive way to create formatted strings. F-strings allow you to embed expressions and variables directly within curly braces {} inside a string, making it easy to combine values and formatting in a readable manner.
Here’s the basic syntax of an f-string:
variable = value formatted_string = f”Some text {variable} more text”
In this syntax, the expression {variable} is evaluated and replaced with the value of the variable within the resulting formatted string.
Here are some examples to illustrate the usage of f-strings:
name = “Alice” age = 25 formatted_string = f”My name is {name} and I am {age} years old.” print(formatted_string)
Output:
My name is Alice and I am 25 years old.
In this example, the values of name and age are directly embedded within the string using f-string syntax.
You can also include expressions and perform operations within the curly braces:
a = 5 b = 3 formatted_string = f”The sum of {a} and {b} is {a + b}.” print(formatted_string)
Output:
The sum of 5 and 3 is 8.
In this example, the expression {a + b} is evaluated and the result is inserted into the formatted string.
F-strings also support various formatting options, such as specifying width, alignment, and precision for numeric values. You can apply these formatting options by adding a colon : followed by a format specifier inside the curly braces.
pi = 3.14159 formatted_number = f”The value of pi is approximately {pi:.2f}” print(formatted_number)
Output:
The value of pi is approximately 3.14
In this example, the format specifier :.2f formats the value of pi as a float with two decimal places.
F-strings offer a powerful and intuitive way to create formatted strings in Python, allowing you to combine values, expressions, and formatting options in a clear and concise manner.
Python Format
In Python, the format() method is a built-in method that allows you to format strings by replacing placeholders with specified values. It provides a flexible and powerful way to format strings using various formatting options.
The basic syntax of the format() method is as follows:
formatted_string = “Some text {} more text”.format(value1, value2, …)
In this syntax, curly braces {} are used as placeholders within the string, and the format() method is called on the string object. The values you want to insert into the placeholders are passed as arguments to the format() method.
Here are some examples to illustrate the usage of the format() method:
name = “Alice” age = 25 formatted_string = “My name is {} and I am {} years old.”.format(name, age) print(formatted_string)
Output:
My name is Alice and I am 25 years old.
In this example, the placeholders {} are replaced with the values of name and age passed to the format() method.
You can also specify the position of the arguments to control their placement within the formatted string:
name = “Alice” age = 25 formatted_string = “My name is {0} and I am {1} years old.”.format(name, age) print(formatted_string)
Output:
My name is Alice and I am 25 years old.
In this example, {0} and {1} indicate the positions of the arguments name and age, respectively.
The format() method also supports various formatting options using format specifiers. For example, you can specify the width, alignment, precision, and data type of the formatted values. Here’s an example:
pi = 3.14159 formatted_number = “The value of pi is approximately {:.2f}”.format(pi) print(formatted_number)
Output:
The value of pi is approximately 3.14
In this example, the format specifier :.2f is used to format the value of pi as a float with two decimal places.
The format() method provides extensive formatting capabilities.
Note that in recent versions of Python (3.6 and above), f-strings (formatted string literals) offer a more concise and readable alternative for string formatting. You might consider using f-strings instead of the format() method for new code.
Python Print Format
In Python, the print() function is used to display output on the console. By default, it prints the specified arguments to the console as separate values, with a space character between them. However, you can also apply formatting to the values passed to print() using various techniques.
String Concatenation:
You can concatenate strings using the + operator to format the output:
name = “Alice” age = 25 print(“My name is “ + name + ” and I am “ + str(age) + ” years old.”)
Output:
My name is Alice and I am 25 years old.
In this example, the strings and variables are concatenated using the + operator, and the str() function is used to convert the age variable to a string.
Comma Separation:
When multiple values are passed as arguments to print(), they are printed with a space character between them by default. You can change this behavior by specifying the sep parameter to define the separator:
name = “Alice” age = 25 print(“My name is”, name, “and I am”, age, “years old.”, sep=“|”)
Output:
My name is|Alice|and I am|25|years old.
In this example, the sep parameter is set to “|” to separate the values with a pipe character instead of a space.
f-strings (formatted string literals):
Starting from Python 3.6, you can use f-strings to easily format the output within the print() function:
name = “Alice” age = 25 print(f”My name is {name} and I am {age} years old.”)
Output:
My name is Alice and I am 25 years old.
In this example, the values are directly embedded within the string using f-string syntax.
These are a few examples of formatting output with the print() function in Python. Depending on your specific requirements, you can choose the method that best suits your needs.
Python String Formatting Cheat Sheet
Certainly! Here’s a cheat sheet for string formatting in Python:
String Interpolation with % Operator (old-style formatting):
name = “Alice” age = 25 print(“My name is %s and I am %d years old.” % (name, age))
Using .format() method (new-style formatting):
name = “Alice” age = 25 print(“My name is {} and I am {} years old.”.format(name, age))
f-strings (formatted string literals):
name = “Alice” age = 25 print(f”My name is {name} and I am {age} years old.”)
Formatting Floating-Point Numbers:
pi = 3.14159 print(“Value of pi: {:.2f}”.format(pi))
Specifying Width and Alignment:
name = “Alice” print(“Name: {:>10}”.format(name)) # Right-aligned, width of 10
Zero Padding for Integers:
number = 42 print(“Number: {:03}”.format(number)) # Zero-padded, width of 3
Formatting Binary and Hexadecimal Numbers:
number = 42 print(“Binary: {:b}”.format(number)) print(“Hexadecimal: {:x}”.format(number))
Using Named Arguments:
name = “Alice” age = 25 print(“My name is {name} and I am {age} years old.”.format(name=name, age=age))
These are just a few examples of string formatting techniques in Python. There are additional formatting options and techniques available.
Python Format Number
In Python, you can format numbers using the format() method, f-strings (formatted string literals), or the % operator. Here are some examples of formatting numbers in Python:
Using the format() method:
number = 123456.789 formatted_number = format(number, “,.2f”) print(formatted_number)
Output:
123,456.79
In this example, the format() method is used to format the number with a comma as a thousands separator and a precision of 2 decimal places.
Using f-strings:
number = 123456.789 formatted_number = f”{number:,.2f}” print(formatted_number)
Output:
123,456.79
In this example, the f-string syntax is used with a format specifier :,.2f to achieve the same formatting result.
Using the % operator (old-style formatting):
number = 123456.789 formatted_number = “%0.2f” % number print(formatted_number)
Output:
123456.79
In this example, the % operator is used with the format specifier %0.2f to format the number with 2 decimal places.
These are just a few examples of how you can format numbers in Python. You can customize the format specifiers to suit your specific requirements when it comes to Python strings format.
Python Strings Format Syntax
Parameter Values
Parameter | Overview |
---|---|
value1, value2… | In Python strings format it is essential that the values can be either a list of values separated by commas, a list of keys and values, or a combination of both. The values can be of any data type. |
A formatted string is returned by the format() method.
Execute
Example
Python strings format allows us to combine strings and numbers with format() method.
- Python Strings Format Methods And Types:
- Python Strings Format – Definition and Usage:
- What Is The Format Of a String In Python?:
- What Is .2f Python Format?:
- What Is %d In String Format?:
- What Does %% s Mean In Python?:
- What Is ___ str __ In Python?:
- What Does \\ S+ S+ Mean In Python?:
- What Does ___ Mean In Python?:
- What Is %s vs d In Python?:
- What Does ‘% d Mean In Python?:
- What Is %R In Python?:
- What Does %C Do In Python?:
- Python Strings Format Float:
- Python Strings Format f:
- Python f-String Format:
- Python f-String:
- Python Format:
- Python Print Format:
- Python String Formatting Cheat Sheet:
- Python Format Number:
- Python Strings Format Syntax:
- Parameter Values:
- The Placeholders:
The Placeholders
Using format(), the passed arguments are formatted and placed in the string where the placeholders {} are:
Numbers can be inserted into strings using the format() method:
Example
In addition to named indices [price], numbered indices [0], and even empty placeholders [] can be used to identify placeholders.
Placing placeholders differently:
Example
Put a fixed point, two-decimal price inside the placeholder:
Example
When we talk about Python strings format then, using the format() method in Python, we can input any number of arguments in the following placeholders:
Execute
Example
Place the arguments in the correct placeholders by using index numbers [0]:
Execute
Example
Formatting Types
Placeholders can be formatted using the following formatting types in Python strings format:
:< |
Execute |
In the available space, align the results to the left |
:> |
Execute |
The result is aligned to the right |
:^ |
Execute |
The result is aligned to the center |
:= |
Execute |
Position the sign to the left |
:+ |
Execute |
Positive or negative results are indicated by a plus sign |
:- |
Execute |
For negative values, use a minus sign |
: |
Execute |
Add a space before positive numbers (and a minus sign before negative numbers). |
:, |
Execute |
Comma as a thousand (1000) separator |
:_ |
Execute |
Separate thousand with an underscore |
:b |
Execute | Binary format |
:c |
Unicode character conversion |
|
:d |
Execute | Decimal format |
:e |
Execute |
Lowercase m, scientific format |
:E |
Execute |
Uppercase M for scientific format |
:f |
Execute |
Point number fix |
:F |
Execute |
Numbers should be in uppercase (INF, NAN). |
:g |
General format |
|
:G |
The general format (using upper case E for scientific notations) |
|
:o |
Execute |
Formatted in octal |
:x |
Execute |
Lowercase hex format |
:X |
Execute |
Formatted in hexadecimal, upper case |
:n |
Format for numbers |
|
:% |
Execute |
Format for percentages |